C Tokens all programs. Token in C language, the Smallest individual units in a program are known as tokens.Therefore, Concept-wise programs are given below. C program of C token is easy to understand by below programs. Depend on the types of tokens there are 6 Tokens:
- Keywords: C language has 32 reserve keywords. All keywords are to be written in a lower-case letter.
- Identifiers: They are use for naming variables, functions, and arrays.
- Constants: In C constants are the identifiers that represent fix values.
- Data Types: Data type specifies the size and type of the value stores in that variable.
- Special Symbols: parenthesis ( ), braces { }, brackets [ ], semicolon ;, etc.
- Operators: It is a symbol that take one or more operands (variables, expressions or values) and operate on them to give an output.
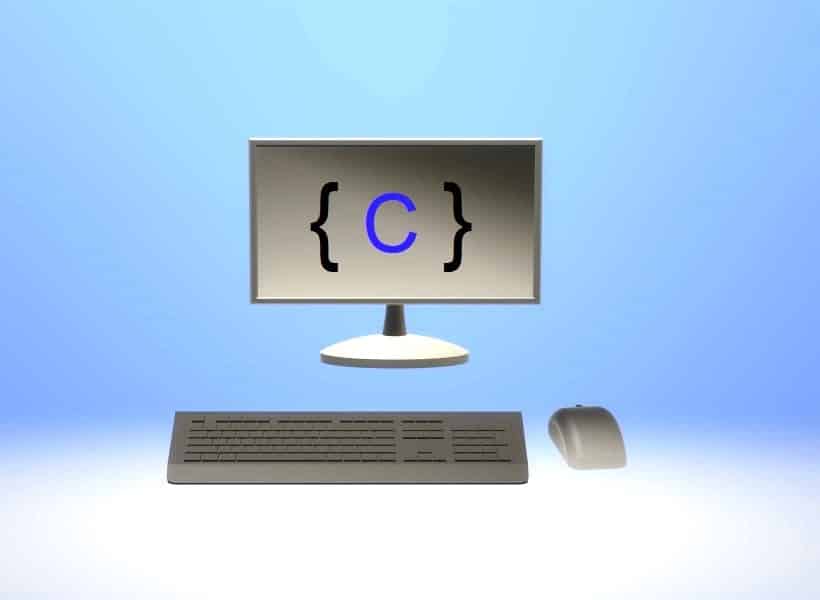
Write a program to reverse a 3 digit number.
#include<stdio.h>
main()
{
int n, n1, rev;
int d1, d2, d3;
n=321;
printf("number = %d\n",n);
n1=n;
d3 = n1%10;
n1=n1/10;
d2=n1%10;
n1=n1/10;
d1=n1;
rev=(d3*100)+(d2*10)+d1;
printf("The reversed number is %d", rev);
}
Output :
number = 321
The reversed number is 123
Write a C program to demonstrate the use of increment operator.
#include<stdio.h>
main()
{
int p = 10, q=20;
q+=++p; //q=q+ ++p
++q;
p++;
p+=q++;
printf("P = %d \nQ = %d",p,q);
}
Output:
P = 44
Q = 33
#include<stdio.h>
int main()
{
int a=5, b=9;
a=++a*++b;
b=b++*a++;
printf("a=%d\nb=%d",a,b);
return 0;
}
Output:
a=61
b=600
C Program to demonstrate the use of shift operators.
#include<stdio.h>
main()
{
int z=20;
printf("%d %d %d \n",z,z<<2>>3,z&100,z|10);
}
Output:
20 10 4 30
Program to illustrate sizeof operator.
#include<stdio.h>
main()
{
int n;
printf("Size of 'float' is %d\n",sizeof(float));
printf("Size of 'n' is %d\n",sizeof(n));
printf("Size of 'char' is %d\n",sizeof(char));
printf("Size of 'A' is %d\n",sizeof('A'));
}
Output:
Size of 'float' is 4
Size of 'n' is 2
Size of 'char' is 1
Size of 'A' is 2
Program to demonstrate the use of symbolic constants.
#include <stdio.h>
#define PRINT(int) printf("int=%d\n",int)
int main()
{
int a=7;
PRINT(a);
return 0;
}
Output:
int=7
#include <stdio.h>
#define SQR(x) x*x
#define CUBE(x) SQR(x)*x
int main()
{
int p,q,r;
p=2;
q=SQR(p++);
r=CUBE(++q);
printf("p=%d\nq=%d\nr=%d",p,q,r);
return 0;
}
Output:
p=4
q=9
r=576
#include<stdio.h>
#define cube(p) (p*p*p)
int main()
{
int p,q;
q=3;
p=cube(++q/++q);
printf("p=%d\nq=%d",p,q);
return 0;
}
Output:
p=0
q=9
Write a program to interchange the values of two variables without using third variable.
#include<stdio.h>
main()
{
int a = 5;
int b = 11;
printf("a = %d\t\nb = %d\n",a,b);
a = a+b;
b = a-b;
a = a-b;
printf("After swapping\na = %d\t\nb = %d",a,b);
}
Output:
a = 5
b = 11
After swapping
a = 11
b = 5
Write a C program to print the amount in coinage analysis.
#include <stdio.h>
int main()
{
int amt, amt1;
int x2000, x500, x200, x100, x50, x20, x10, x5, x1;
amt=4285;
amt1=amt;
printf("Amount=%d\n", amt);
x2000=amt1/2000;
amt1=amt1%2000;
x500=amt1/500;
amt1=amt1%500;
x200=amt1/200;
amt1=amt1%200;
x100=amt1/100;
amt1=amt1%100;
x50=amt1/50;
amt1=amt1%50;
x20=amt1/20;
amt1=amt1%20;
x10=amt1/10;
amt1=amt1%10;
x5=amt1/5;
amt1=amt1%5;
x1=amt1/1;
amt1=amt1%1;
printf("Notes\tNo of Notes\n");
printf("-----\t-----------\n");
printf("2000\t%d\n",x2000);
printf("500\t%d\n",x500);
printf("200\t%d\n",x200);
printf("100\t%d\n",x100);
printf("50\t%d\n",x50);
printf("20\t%d\n",x20);
printf("10\t%d\n",x10);
printf("5\t%d\n",x5);
printf("1\t%d\n",x1);
}
Output:
Amount=4285
Notes No of Notes
----- -----------
2000 2
500 0
200 1
100 0
50 1
20 1
10 1
5 1
1 0
C Program which accepts a decimal number and convert it into hexadecimal number.
#include <stdio.h>
void main()
{
int a;
printf("Enter the number:");
scanf("%d", &a);
printf("\nYou entered decimal number: %d",a);
printf("\nEquivalent hexadecimal number: %x",a);
}
Output:
Enter the number: 11
You entered decimal number: 11
Equivalent hexadecimal number: b
Program to illustrate the use of character datatypes.
#include<stdio.h>
main()
{
char c1='a',c2;
c2=c1+1;
printf("c1 = %c nc2 = %c",c1,c2);
}
Output : c1 = a
c2 = b
Program to calculate number of months and days.
//Program to calculate number of months and days
#include<stdio.h>
main()
{
int m,d=165;
m=165/30;
d=d%30;
printf("No. of months = %d \n No. of days = %d",m,d);
}
Output :
No. of months = 5
No. of days = 15
Write a program to find the greatest number out of two numbers using a conditional operator.
#include<stdio.h>
main()
{
int a=1, b=3, c;
c= (a>b ? a : b);
printf("The greatest number is %d", c);
}
Output :
The greatest number is 3