The structure is a collection of logically related data items of different data types group together under a single name. It is similar to records. However, the records contain different fields, the data items that make a structure are known as its members or fields. Structures and Unions C program contains concept-oriented programs
Syntax:
struct structure_name
{
data_type member1;
data_type member2;
};
Unions are like Structures are the user-define data type and somewhat similar to structures as they also contain members of different data types. Thus, All members in the Union share the same storage area in the computer memory while each member in the Structure is assigning its own unique storage area. Since the members of the union share the same memory, only one member can be active at a time. Structures and Unions C programs.
Although, Unions are useful as they efficiently use the computer’s memory. They are useful in cases where you have to include multiple members provide the values need not be assign to all the members at any one time.
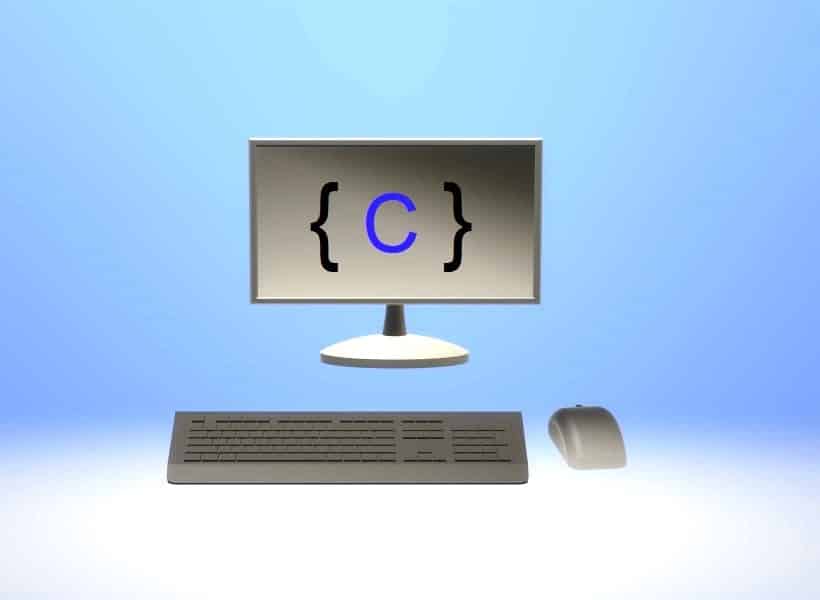
Write a program to accept name, address and mobile number of employee using the structure and display it.
#include<stdio.h>
struct e; // Structure Datatype Declaration
void main()
{
struct e
{
char name[50];
int mobile;
char address[100];
}; // Structure Definition
struct e e1; // Structure Variable Declaration
printf("Enter Name of the Employee: ");
scanf("%s",e1.name); // Accessing Name
printf("\nEnter Mobile number of the Employee: ");
scanf("%d",&e1.mobile);
printf("\nEnter Address of the Employee: ");
scanf("%s",e1.address);
printf("\nName of the Employee: %s",e1.name);
printf("\nMobile of the Employee: %d",e1.mobile);
printf("\nAddress of the Employee: %s",e1.address);
}
Output:
Enter Name of the Employee: Codeforever
Enter Mobile number of the Employee: 000100121
Enter Address of the Employee: Code
Name of the Employee: Codeforever
Mobile of the Employee: 100121
Address of the Employee: Code
Program to find out duration between the time
#include<stdio.h>
struct TIMEDURATION
{
int hh, mm, ss;
char dn[3];
};
void main()
{
struct TIMEDURATION tm1, tm2, tm3;
int q;
printf("Enter first time for 12 hr clock: \n");
scanf("%d%d%d", &tm1.hh, &tm1.mm, &tm1.ss);
printf("am or pm?\n");
scanf("%s", tm1.dn);
printf("Enter second time for 12 hr clock: \n");
scanf("%d%d%d", &tm2.hh, &tm2.mm, &tm2.ss);
printf("am or pm?\n");
scanf("%s", tm2.dn);
q=tm2.hh;
if(strcmp(tm1.dn, tm2.dn)!=0)
{
tm2.hh+=12;
}
tm3.hh=tm2.hh-tm1.hh;
if(tm2.mm>tm1.mm)
tm3.mm=tm2.mm-tm1.mm;
else
{
tm3.hh--;
tm3.mm=(tm2.mm+60)-tm1.mm;
}
if(tm2.ss>tm1.ss)
tm3.ss=tm2.ss-tm1.ss;
else
{
tm3.mm--;
if(tm3.mm==-1)
{
tm3.mm=0;
tm3.hh--;
}
tm3.ss=(tm2.ss+60)-tm1.ss;
}
printf("Time 1 is: %2d:%2d:%2d %s\n", tm1.hh, tm1.mm, tm1.ss, tm1.dn);
printf("Time 2 is: %2d:%2d:%2d %s\n", q, tm2.mm, tm2.ss, tm2.dn);
printf("Duration is: %d hours, %d minutes, %d seconds", tm3.hh, tm3.mm, tm3.ss);
}
Output:
Enter first time for 12 hr clock:
10 12 35
am or pm?
am
Enter second time for 12 hr clock:
09 32 15
am or pm?
pm
Time 1 is: 10:12:35 am
Time 2 is: 9:32:15 pm
Duration is: 11 hours, 19 minutes, 40 seconds
Accept Basic pay, dearness allowance, travelance allowance and house rent allowance of n employee and calculate the salary of employees.
DA is 35% od basic pay
TA is 15% of basic pay
HRA is 20% of basic pay
#include<stdio.h>
struct employee
{
char name[20];
double b,da,ta,hra,netsalary;
};
void main()
{
struct employee emp[100];
int i,n;
double t;
printf("Total Employee: ");
scanf("%d",&n);
for(i=0;i<n;i++)
{
printf("\nEnter the Name of Employee: ");
scanf("%s",emp[i].name);
printf("\nEnter the Basic Salary: ");
scanf("%f",&t);
emp[i].b=t;
emp[i].da=emp[i].b*0.35;
emp[i].ta=emp[i].b*0.15;
emp[i].hra=emp[i].b*0.20;
emp[i].netsalary=emp[i].b+emp[i].da+emp[i].ta+emp[i].hra;
}
printf("\nName Basic Pay DA TA HRA Net Salary");
for(i=0;i<n;i++)
{
printf("\n%-8s %5.2lf %5.2lf %5.2lf %5.2lf %7.2lf",emp[i].b,emp[i].da,emp[i].ta,emp[i].hra,emp[i].netsalary);
}
}
Output:
Write a program to initialize values to all members of the structure.
#include<stdio.h>
struct item;
void main()
{
struct item
{
char name[50];
int qty;
float rate,price;
}i={"Pencil",3,22.25,52.0};
printf("\nThe Name of the Item: %s",i.name);
printf("\nQuantity of the Item: %d",i.qty);
printf("\nRate of the Item: %f",i.rate);
printf("\nPrice of the Item: %f",i.price);
}
Output:
The Name of the Item: Pencil
Quantity of the Item: 3
Rate of the Item: 22.250000
Price of the Item: 52.000000
Program to find out duration between dates.
#include<stdio.h>
struct DATEDURATION
{
int dd, mm, yy;
};
int mon[12]={31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
void main()
{
struct DATEDURATION dt1, dt2, dt3;
int days;
printf("Enter first date: \n");
scanf("%d/%d/%d", &dt1.dd, &dt1.mm, &dt1.yy);
printf("Enter second date: \n");
scanf("%d/%d/%d", &dt2.dd, &dt2.mm, &dt2.yy);
if((dt2.yy%4)==0)
mon[1]=29;
else
mon[1]=28;
dt3.yy=dt2.yy-dt1.yy;
if(dt2.mm>dt1.mm)
dt3.mm=dt2.mm-dt1.mm;
else
{
dt3.yy--;
if(dt3.yy==-1)
{
printf("Invalid dates\n Second date should greater");
return;
}
dt3.mm=(dt2.mm+12)-dt1.mm;
}
if(dt2.dd>dt1.dd)
dt3.dd=dt2.dd-dt1.dd;
else
{
dt3.mm--;
if(dt3.mm<=0)
{
dt3.mm=0;
dt3.yy--;
if(dt3.yy==-1)
{
printf("Invalid dates\n Second date should greater");
return;
}
dt3.dd=(dt2.dd+mon[dt2.mm-1])-dt1.dd;
}
}
printf("\nDate 1:%d/%d/%d",dt1.dd,dt1.mm,dt1.yy);
printf("\nDate 2:%d/%d/%d",dt2.dd,dt2.mm,dt2.yy);
printf("\nDuration: %d days, %d months, and %d years",dt3.dd,dt3.mm,dt3.yy);
Output:
Enter first date:
12/2/2001
Enter second date:
23/03/2006
Date 1:12/2/2001
Date 2:23/3/2006
Duration: 11 days, 1 months, and 5 years
Write a program to calculate average marks of the students, where student has three subjects.
#include<stdio.h>
struct student
{
char name[20];
int m1,m2,m3;
float average;
};
void main()
{
struct student s1,s2;
printf("Enter the name of student: \n");
scanf("%s",s1.name);
printf("Enter the marks of 3 subjects of the student: \n");
scanf("%d%d%d",&s1.m1,&s1.m2,&s1.m3);
s1.average=(s1.m1+s1.m2+s1.m3)/3;
printf("Enter the name of the student: \n");
scanf("%s",s2.name);
printf("Enter the marks of 3 subjects of the student: \n");
scanf("%d%d%d",&s2.m1,&s2.m2,&s2.m3);
s2.average=(s2.m1+s2.m2+s2.m3)/3;
printf("Name of the student: %s\n",s1.name);
printf("Marks of the student: %d %d %d\n",s1.m1,s1.m2,s1.m3);
printf("Average of the marks: %f\n",s1.average);
printf("Name of the student: %s\n",s2.name);
printf("Marks of the student: %d %d %d \n",s2.m1,s2.m2,s2.m3);
printf("Average of the marks: %f",s2.average);
}
Output:
Enter the name of student:
Code
Enter the marks of 3 subjects of the student:
68 98 45
Enter the name of the student:
Forever
Enter the marks of 3 subjects of the student:
89 95 75
Name of the student: Code
Marks of the student: 68 98 45
Average of the marks: 70.000000
Name of the student: Forever
Marks of the student: 89 95 75
Average of the marks: 86.000000
Program to find out whether time is valid or not.
#include<stdio.h>
struct time
{
int hh,mm,ss;
char dn[3];
};
void main()
{
struct time t1;
printf("\nEnter Time of 12 hour clock\n");
scanf("%d:%d:%d %s",&t1.hh,&t1.mm,&t1.ss,t1.dn);
if(t1.hh<=12)
if(t1.mm<60)
if(t1.ss<60)
{
printf("\nTime %d:%d:%d %s is valid",t1.hh,t1.mm,t1.ss,t1.dn);
return;
}
printf("\nTime %d:%d:%d %s is not valid",t1.hh,t1.mm,t1.ss,t1.dn);
}
Output:
Enter Time of 12 hour clock
05:51:34 am
Time 5:51:34 am is valid