In C, A function is a name, self-contain block of statements that perform a specific, well-define task and may return a value to the calling program. It is reference by a name and is invoke (or calls) by this name. It is self-contained as it can have its own variables and constants to be use only within the function in C. A function performs a specific tasks as it define a discrete job to perform as a part of the overall program. A function may or may not return a value to the calling program. All programs below are related to functions concept various programs are depend on the terms of functions. Therefore, you get conceptual-base learning here. Follow the below programs for practice.
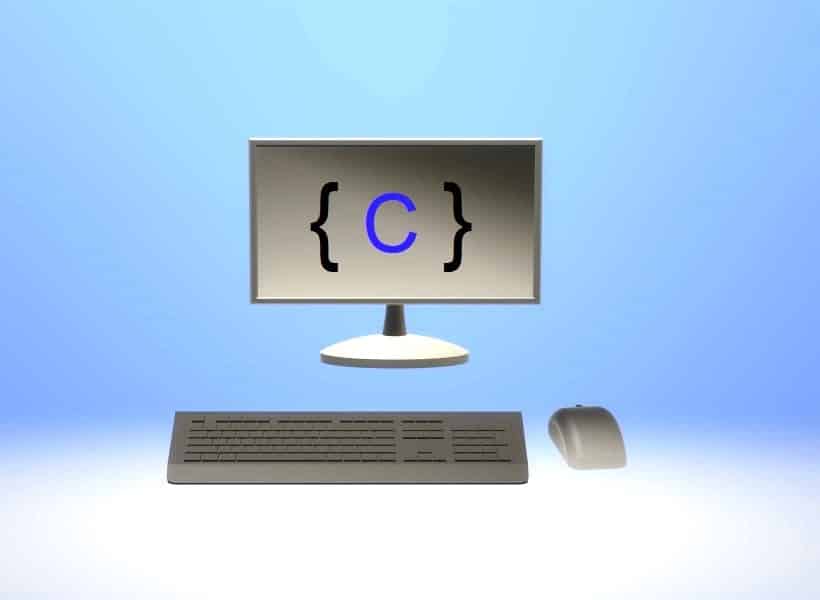
Program to calculate ‘x’ using recursion
#include<stdio.h>
int main()
{
int x, y, s;
printf("Enter the value of x and y: ");
scanf("%d %d", &x, &y);
s= power(x, y);
printf("\n%d^%d = %d", x, y, s);
return 0;
}
int power(int s, int n)
{
int p=1, i;
if(n==1)
return s;
else
p=s*power(s, n-1);
return p;
}
Output:
Enter the value of x and y: 4 6
4^6 = 4096
Write a C program to print odd numbers up to n using recursion.
#include<stdio.h>
void odd(int a, int n)
{
if(a<=n)
{
printf("\n%d ",a);
odd(a+2,n);
}
return;
}
void main()
{
int n;
printf("Enter the value of n : ");
scanf("%d",&n);
odd(1,n);
}
Output:
Enter the value of n : 23
1
3
5
7
9
11
13
15
17
19
21
23
Program to print and add n even numbers using recursion.
#include<stdio.h>
int main()
{
int a, n;
printf("Enter the value of n \n");
scanf("%d", &n);
a=even(0, 1, n);
printf("\b = %d", a);
}
int even(int e, int cnt, int n)
{
static int sum=0;
if(cnt<=n)
{
sum+=e;
printf("%d + ", e);
even(e+2, cnt+1, n);
}
else
return sum;
}
Output:
Enter the value of n 25
0 + 2 + 4 + 6 + 8 + 10 + 12 + 14 + 16 + 18 + 20 + 22 + 24 + 26 + 28 + 30 + 32 + 34 + 36 + 38 + 40 + 42 + 44 + 46 + 48 + = 600
Program to print and solve the following series using recursion.
x + x^3/3! + x^5/5! —– x^n/n!
#include <stdio.h>
void main()
{
int x, n;
double s;
printf("Enter the value of x and n");
scanf("%d%d", &x, &n);
series(1, x, n);
}
int fact(int p)
{
int f=1, i;
if(p==1)
return 1;
else
f=p*fact(p-1);
return f;
}
int pow(int a, int n)
{
int p=1, i;
if(n==1)
return a;
else
p=a*pow(a, n-1);
return p;
}
double term(int x, int a)
{
return ((double)pow(x, a)/fact(a));
}
void series(int a, int x, int n)
{
static double sum=0.0;
if(a<=n)
{
printf("%d^%d/%d!=%f)+", x, a, a, term(x, a));
sum+=term(x, a);
series(a+2, x, n);
}
else
printf("\b=%lf", sum);
}
Output:
Enter the value of x and n 3 9
3^1/1!=3.000000)+3^3/3!=4.500000)+3^5/5!=2.025000)+3^7/7!=0.433929)+3^9/9!=0.054241)=10.013170
C Program to calculate GCD of two numbers.
#include<stdio.h>
void main()
{
int x, y;
printf("Enter two numbers: \n");
scanf("%d%d", &x, &y);
if(x<y)
printf("GCD of %d and %d is: %d", x, y, gcd(x, y));
else
printf("GCD of %d and %d is: %d", x, y, gcd(x, y));
}
int gcd(int p, int q)
{
int rem;
rem=q%p;
while(rem!=0)
{
q=p;
p=rem;
rem=q%p;
}
return 0;
}
Output:
Enter two numbers: 2356 23
GCD of 2356 and 23 is: 0
Program to convert decimal number to binary number using function.
#include<stdio.h>
void main()
{
int no;
printf("Enter any number: \n");
scanf("%d", &no);
d2b(no);
}
void d2b(int no)
{
int x[20], y[20], p, q, r;
p=0;
while(no>0)
{
x[p]=no%2;
p++;
no=no/2;
}
r=p-1;
for(q=0;q<p;q++)
{
y[q]=x[r];
printf("%d", y[q]);
r--;
}
}
Output:
Enter any number:
12
1100
Program to write a recursive function to display first n Fibonacci numbers.
#include<stdio.h>
void main()
{
int n, p=0, x;
printf("Numbers to be generate in Fibonacci series: \n");
scanf("%d", &n);
printf("Fibonacci series:\n");
for(x=1;x<=n;x++)
{
printf("%d ", Fibonacci(p));
p++;
}
return 0;
}
int Fibonacci(int n)
{
if(n==0)
return 0;
else if(n==1)
return 1;
else
return(Fibonacci(n-1)+Fibonacci(n-2));
}
Output:
Numbers to be generate in Fibonacci series: 15
Fibonacci series:
0 1 1 2 3 5 8 13 21 34 55 89 144 233 377
Recursive program to add first N numbers.
#include<stdio.h>
int main()
{
int n, add;
printf("Enter value of n: \n");
scanf("%d", &n);
add=recursum(n);
printf("Addition of first %d numbers is: %d", n, add);
}
int recursum(int n)
{
if(n==0)
return n;
else
return n+recursum(n-1);
}
Output:
Enter value of n:
10
Addition of first 10 numbers is: 55
Program to calculate LCM of two numbers.
#include<stdio.h>
int main()
{
int x, y, s;
printf("Enter two numbers:\n");
scanf("%d%d", &x, &y);
if(x>y)
s=LCM(x, y);
else
s=LCM(x, y);
printf("LCM of %d and %d is: %d", x, y, s);
return 0;
}
int LCM(int m, int n)
{
static int temp=1;
if(temp%n==0 && temp%m==0)
return temp;
temp++;
LCM(m, n);
return temp;
}
Output:
Enter two numbers: 7 5
LCM of 7 and 5 is: 35
Program to calculate GCD of two numbers using recursion.
#include<stdio.h>
void main()
{
int x, y, divisor;
printf("Enter two numbers: \n");
scanf("%d%d", &x, &y);
divisor=gcd(x, y);
printf("GCD of %d and %d is: %d", x, y, divisor);
getch();
}
gcd(int x, int y)
{
if(y<=x && x%y==0)
return y;
if(x<y)
return gcd(y, x);
else
return gcd(y, x%y);
}
Output:
Enter two numbers:
326 6
GCD of 326 and 6 is: 2