Flow Control Structures in C: Decision-making is to see whether a particular condition has occurred or not and then direct the computer to execute certain statements accordingly. Hence, C Control Structures are mainly two types Branching and Lopping.
Branching : When a program breaks the sequential flows and jumps to another part of the code.
There are two types of Branching :
- Conditional Branching : This statements checks the condition first and then control is transfers to another part of the code.
- Unconditional Branching : This statements do not check the condition and the control is transferred to another part of the code.
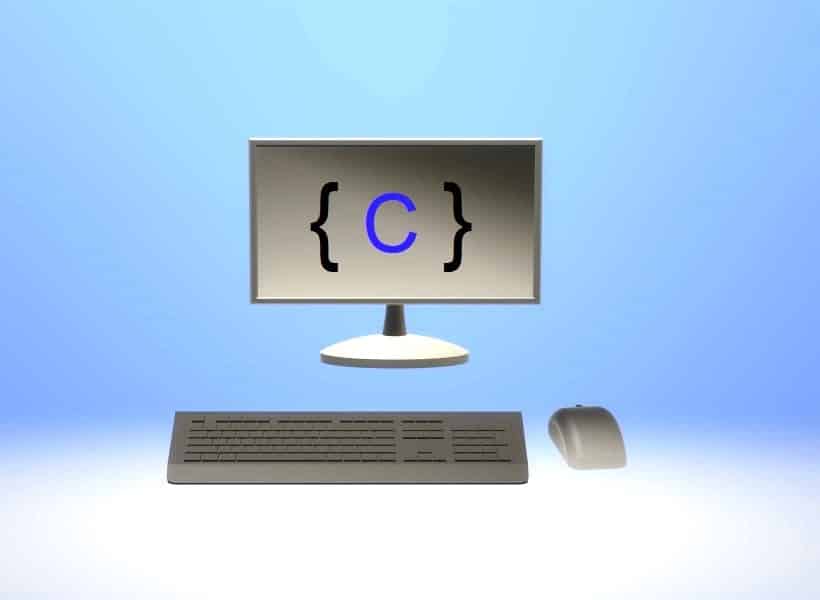
C Program to print alphabets in a desirable manner.
#include <stdio.h>
void main()
{
int p=1, q, r, a=71, blanks=0, value;
while(p<=7)
{
q=65;
value=a;
while(q<=value)
{
printf("%c", q);
q++;
}
if(p==1)
value--;
r=1;
while(r<=blanks)
{
printf(" ");
r++;
}
blanks=2*p-1;
while(value>=65)
{
printf("%c", value);
value--;
}
printf("\n");
a--;
p++;
}
}
Output:
ABCDEFGFEDCBA
ABCDEF FEDCBA
ABCDE EDCBA
ABCD DCBA
ABC CBA
AB BA
A A
C Program to accept 10 numbers and printing sum of odd and even numbers.
#include<stdio.h>
void main()
{
int x[10], p, e=0, o=0;
printf("Enter any ten numbers: \n");
for(p=0;p<10;p++)
scanf("%d", &x[p]);
for(p=0;p<10;p++)
{
if(x[p]%2==0)
e=e+x[p];
else
o=o+x[p];
}
printf("Sum of odd is: %d\n", o);
printf("Sum of even is: %d", e);
}
OUTPUT:
Enter any ten numbers:
4 76 21 53 82 49 35 64 93 17
Sum of odd is: 268
Sum of even is: 226
Write a program to evaluate the sum of the series for n-terms:
1^2/1! + 2^2/2! + 3^2/3! +……+ n^2/n!
#include <stdio.h>
void main()
{
int n, p, q, f, t, sum;
printf("Enter the number\n");
scanf("%d", &n);
printf("The series: ", n);
for(p=1; p<=n; p++)
{
printf("%d^2/%d! + ", p, p);
f=1;
for(q=1; q<=p; q++)
f*=q;
t=(p*p)/f;
sum+=t;
}
printf("\b\nSum of series: %d", sum);
}
Output:
Enter the number
10
The series: 1^2/1! + 2^2/2! + 3^2/3! + 4^2/4! + 5^2/5! + 6^2/6! + 7^2/7! + 8^2/8! + 9^2/9! + 10^2/10! +
Sum of series: 4200948
Write a program to compute N terms of the following series:
1 – x^2/2! + x^4/4! + x^6/6! + ….. + x^n/n!
#include <stdio.h>
void main()
{
int x, n, s, f, t, p, q, sign=-1;
float sum=1.0;
printf("Enter the last term n: \n");
scanf("%d", &n);
printf("Enter the value of x: \n");
scanf("%d", &x);
printf("The series =1");
for(p=2; p<=n; p+=2)
{
printf("%d*x^%d/%d! + ", sign, p, p);
f=1;
for(q=1; q<=p; q++)
f*=q;
s=1;
for(q=1; q<=p; q++)
s*=x;
t=s/f*sign;
sum+=t;
sign*=-1;
}
printf("\nSum of series: %f", sum);
}
Output:
Enter the last term n:
5
Enter the value of x:
2
The series =1-1*x^2/2! + 1*x^4/4! +
Sum of series: -1.000000
Write a program to compute first N terms of the following series:
x – x^3/3! + x^5/5! + x^7/7! + ….. + x^n/n!
#include <stdio.h>
void main()
{
int x, n, s, f, term, p, q, sign=1;
float sum=0.0;
printf("Enter the last term n: \n");
scanf("%d", &n);
printf("Enter the value of x: \n");
scanf("%d", &x);
printf("The series =1");
for(p=1; p<=n; p+=2)
{
printf("%d*x^%d/%d! + ", sign, p, p);
f=1;
for(q=1; q<=p; q++)
f*=q;
s=1;
for(q=1; q<=p; q++)
s*=x;
term=s/f*sign;
sum+=term;
sign*=-1;
}
printf("\nSum of series: %f", sum);
}
Output:
Enter the last term n: 30
Enter the value of x: 10
The series =11*x^1/1! + -1*x^3/3! + 1*x^5/5! + -1*x^7/7! + 1*x^9/9! + -1*x^11/11! + 1*x^13/13! + -1*x^15/15! + 1*x^17/17! + -1*x^19/19! + 1*x^21/21! + -1*x^23/23! + 1*x^25/25! + -1*x^27/27! + 1*x^29/29! +
Sum of series: 1432.000000
Write a C program to find the sum of first ‘n’ terms of the following series:
1/(x+1) + 2/(x+1)^2 + 3/(x+1)^3 +….. + n/(x+1)^n.
#include <stdio.h>
void main()
{
int x, n, s, p, q;
float t, sum = 0.0;
printf("Enter the last term n: \n");
scanf("%d", &n);
printf("Enter the value of x: \n");
scanf("%d", &x);
printf("The series: ");
for(p=1; p<=n; p++)
{
printf("%d/(x+1)^%d + ", p,p);
s=1;
for(q=1; q<=p; q++)
s*=(x+1);
t=(float)p/s;
sum+=t;
}
printf("\nSum of series: %f", sum);
}
Output:
Enter the last term n: 5
Enter the value of x: 2
The series: 1/(x+1)^1 + 2/(x+1)^2 + 3/(x+1)^3 + 4/(x+1)^4 + 5/(x+1)^5 +
Sum of series: 0.736626
Write a program to print number by skipping zeros.
#include <stdio.h>
int main()
{
int a, d, t, nn=0;
printf("Enter the number: \n");
scanf("%d", &a);
t=a;
do
{
nn=nn*10+(t%10);
t=t/10;
}
while(t!=0);
t=nn;
nn=0;
do
{
d=t%10;
if(d!=0)
nn=nn*10+d;
t=t/10;
}
while(t!=0);
printf("Original number: %d\n", a);
printf("Number after skipping zero: %d", nn);
return 0;
}
Output:
Enter the number: 20072
Original number: 20072
Number after skipping zero: 272
C Program to print LCM of two numbers.
#include <stdio.h>
int main()
{
int p, q, temp, pro, rem, lcm;
printf("Enter two numbers\n");
scanf("%d %d", &p, &q);
pro=p*q;
do
{
if(p<q)
{
temp=p;
p=q;
q=temp;
}
rem=p%q;
if(rem==0)
{
lcm=pro/q;
}
else
{
p=q;
q=rem;
}
}
while(rem!=0);
printf("LCM : %d", lcm);
return 0;
}
Output:
Enter two numbers 2 5
LCM : 10
Program to sort given list of numbers in descending order.
#include <stdio.h>
int main()
{
int arr[30], p,q, n, temp;
printf("Enter the number of elements you want to sort: \n");
scanf("%d", &n);
printf("Enter the elements: \n");
for(p=0;p<n;p++)
scanf("%d", &arr[p]);
for(p=0;p<n-1;p++)
{
for(q=p+1;q<n;q++)
{
if(arr[p]>arr[q])
{
temp=arr[p];
arr[p]=arr[q];
arr[q]=temp;
}
}
}
printf("Elements after sorting: \n");
for(p=n-1;p>=0;p--)
printf("%d ", arr[p]);
return 0;
}
Output:
Enter the number of elements you want to sort: 5
Enter the elements: 2 5 3 4 1
Elements after sorting:
5 4 3 2 1
Program to display how many non zero integers entered.
#include <stdio.h>
int main()
{
int a, cnt=0;
do
{
printf("Enter number: \n");
scanf("%d", &a);
cnt++;
}
while(a!=0);
printf("Total number of non-zero integers: %d\n", cnt-1);
return 0;
}
Output:
Enter number: 20
Enter number: 55
Enter number: 12
Enter number: 0
Total number of non-zero integers: 3
C Program to display the following pattern:
A1
B2 C3
D4 E5 F6
G7 H8 I9 J10
#include<stdio.h>
void main()
{
int f=1, a, p, q;
char c='A';
printf("How many lines: \n");
scanf("%d", &a);
for(p=0;p<a;p++)
{
for(q=0;q<=p;q++)
{
printf("%c%d ", c, f);
f++;
c++;
}
printf("\n");
}
}
Output: How many lines: 4 A1 B2 C3 D4 E5 F6 G7 H8 I9 J10
Write a program to display n odd numbers, It demonstrates continue and break statements.
#include<stdio.h>
main()
{
int n,i=0;
printf("\n Enter Last Odd Number : ");
scanf("%d",&n);
printf("\n Odd Numbers : \0");
while(1)
{
if(i%2==0)
{
i++;
continue;
}
if(i>n)
break;
printf("%d ",i);
i++;
}
}
Output :
Enter Last Odd Number : 25
Odd Numbers : 1 3 5 7 9 11 13 15 17 19 21 23 25