Pointers are the variables that contain the address of another variable within the memory.
Every data item in the computer is stored in the memory in one or more adjacent locations depending upon its type. The computer’s memory is a sequential collection of the storage cells. Each cell is known as a byte and has a number called address associated with it. They are name in a serial manner, starting from zero. Therefore, Various examples of pointers program given below.
Declaration:
Syntax : data_type *ptr;
where data_type: Data type of the pointer’s object.
* (asterisk): It indicates that this variable is a pointer.
ptr : Name of the pointer variable.
Initialization:
Use of the address of & operator as a prefix to the variable name assigns its address to the pointer.
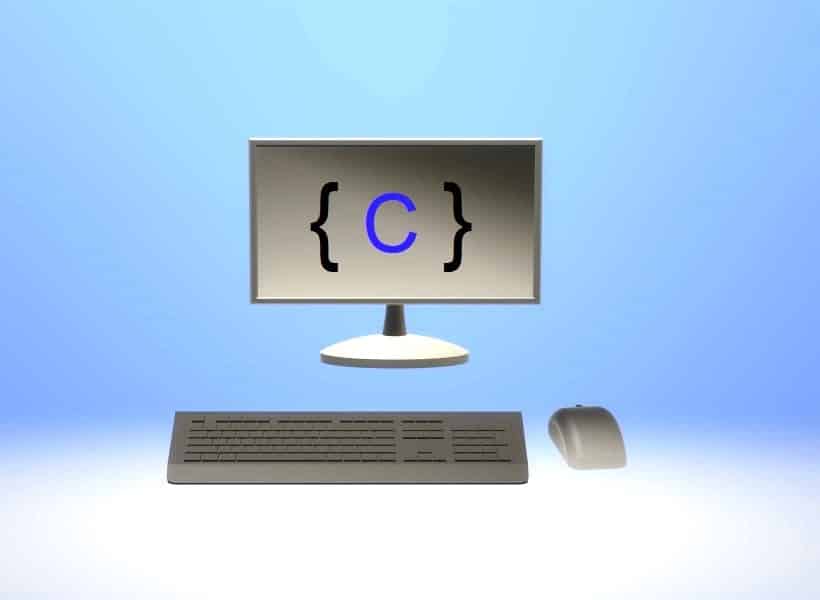
C program to print the address of variable along with it’s value.
#include <stdio.h>
void main()
{
char c='S';
float f=12.9;
int i=351;
printf("Name: c Value: %c Address: %X Type: char\n", c, &c);
printf("Name: f Value: %f Address: %X Type: float\n", f, &f);
printf("Name: i Value: %d Address: %X Type: int\n", i, &i);
}
OUTPUT:
Name: c Value: S Address: 6D6BC96F Type: char
Name: f Value: 12.900000 Address: 6D6BC968 Type: float
Name: i Value: 351 Address: 6D6BC964 Type: int
C Program to demonstrate the pointers.
#include <stdio.h>
void main()
{
char c='S', *cp;
float f=25.7, *fp;
int i=539, *ip;
cp=&c;
fp=&f;
ip=&i;
printf("Value of C: %c\t\t\tcp=%X\t\t*cp=%X\t\t\t& of cp=%X\n", c, cp, *cp, &cp);
printf("Value of F: %f fp=%X\t\t*fp=%X\t& of fp=%X\n", f, fp, *fp, &fp);
printf("Value of I: %d\t\t\tip=%X\t\t*ip=%X\t\t\t& of ip=%X\n\n", i, ip, *ip, &ip);
(*cp)--;
f+=250.7;
(*ip)--;
printf("Value of C: %c\t\t\tcp=%X\t\t*cp=%X\t\t\t& of cp=%X\n", c, cp, *cp, &cp);
printf("Value of F: %f fp=%X\t\t*fp=%X\t& of fp=%X\n", f, fp, *fp, &fp);
printf("Value of I: %d\t\t\tip=%X\t\t*ip=%X\t\t\t& of ip=%X\n", i, ip, *ip, &ip);
}
OUTPUT:
Value of C: S cp=FFFFEBAF *cp=53 & of cp=FFFFEBA0
Value of F: 25.700001 fp=FFFFEB9C *fp=FFFFEB90 & of fp=0
Value of I: 539 ip=FFFFEB8C *ip=21B & of ip=FFFFEB80
Value of C: R cp=FFFFEBAF *cp=52 & of cp=FFFFEBA0
Value of F: 276.399994 fp=FFFFEB9C *fp=FFFFEB90 & of fp=0
Value of I: 538 ip=FFFFEB8C *ip=21A & of ip=FFFFEB80
Write program to show pointer arithmatic.
// By Code Forever
#include <stdio.h>
void main()
{
char c='S', *cp;
float f=12.5, *fp;
int i=486, *ip;
long l=517, *lp;
double d=10.99, *dp;
cp=&c;
fp=&f;
ip=&i;
lp=&l;
dp=&d;
printf("Char Float int Long Double\n");
printf("%8X %8X %8X %8X %8X\n", cp, fp, ip, lp, dp);
cp++;
fp++;
ip++;
lp++;
dp++;
printf("%8X %8X %8X %8X %8X\n", cp, fp, ip, lp, dp);
cp+=12;
fp+=12;
ip+=12;
lp+=12;
dp+=12;
printf("%8X %8X %8X %8X %8X\n", cp, fp, ip, lp, dp);
cp--;
fp--;
ip--;
lp--;
dp--;
printf("%8X %8X %8X %8X %8X\n", cp, fp, ip, lp, dp);
cp-=12;
fp-=12;
ip-=12;
lp-=12;
dp-=12;
printf("%8X %8X %8X %8X %8X", cp, fp, ip, lp, dp);
}
OUTPUT:
Char Float Int Long Double
FFFFEB87 FFFFEB80 FFFFEB7C FFFFEB70 FFFFEB68
FFFFEB88 FFFFEB84 FFFFEB80 FFFFEB78 FFFFEB70
FFFFEB94 FFFFEBB4 FFFFEBB0 FFFFEBD8 FFFFEBD0
FFFFEB93 FFFFEBB0 FFFFEBAC FFFFEBD0 FFFFEBC8
FFFFEB87 FFFFEB80 FFFFEB7C FFFFEB70 FFFFEB68
Write program to show pointer arithmetic (using for loop).
#include <stdio.h>
void main()
{
int c='S', *cp;
float f=12.5, *fp;
int i=486, *ip;
long l=517, *lp;
double d=10.99, *dp;
cp=&c;
fp=&f;
ip=&i;
lp=&l;
dp=&d;
printf("Char Float Int Long Double\n");
printf("%8X %8X %8X %8X %8X\n", cp, fp, ip, lp, dp);
for(i=0;i<3;i++)
{
cp++;
fp++;
ip++;
lp++;
dp++;
printf("%8X %8X %8X %8X %8X\n", cp, fp, ip, lp, dp);
}
for(i=0;i<3;i++)
{
cp--;
fp--;
ip--;
lp--;
dp--;
printf("%8X %8X %8X %8X %8X\n", cp, fp, ip, lp, dp);
}
}
OUTPUT:
Char Float Int Long Double
FFFFEB84 FFFFEB80 FFFFEB7C FFFFEB70 FFFFEB68
FFFFEB88 FFFFEB84 FFFFEB80 FFFFEB78 FFFFEB70
FFFFEB8C FFFFEB88 FFFFEB84 FFFFEB80 FFFFEB78
FFFFEB90 FFFFEB8C FFFFEB88 FFFFEB88 FFFFEB80
FFFFEB8C FFFFEB88 FFFFEB84 FFFFEB80 FFFFEB78
FFFFEB88 FFFFEB84 FFFFEB80 FFFFEB78 FFFFEB70
FFFFEB84 FFFFEB80 FFFFEB7C FFFFEB70 FFFFEB68
Program to show pointer to pointer.
#include <stdio.h>
void main()
{
int c='S', *cp, **pcp;
float f=12.5, *fp, **pfp;
int i=486, *ip, **pip;
long l=517, *lp, **plp;
double d=10.99, *dp, **pdp;
cp=&c;
fp=&f;
ip=&i;
lp=&l;
dp=&d;
pcp=&cp;
pfp=&fp;
pip=&ip;
plp=&lp;
pdp=&dp;
printf("Data type Address of pointer Address of value Value\n");
printf("**p \t *p \t p\n");
printf("Character %8X %10X %c\n", pcp, cp, c);
printf("Float %8X %10X %f\n", pfp, fp, f);
printf("Integer %8X %10X %d\n", pip, ip, i);
printf("Long %8X %10X %ld\n", plp, lp, l);
printf("Double %8X %10X %ld\n", pdp, dp, d);
}
OUTPUT:
Data type Address of pointer Address of value Value
**p *p p
Character 61FF04 61FF08 S
Float 61FEFC 61FF00 12.500000
Integer 61FEF4 61FEF8 486
Long 61FEEC 61FEF0 517
Double 61FEDC 61FEE0 1202590843
Program to show pointer to pointer (using arithmetic operation).
#include <stdio.h>
void main()
{
int c='S', *cp, **pcp;
float f=12.5, *fp, **pfp;
int i=486, *ip, **pip;
long l=517, *lp, **plp;
double d=10.99, *dp, **pdp;
cp=&c;
fp=&f;
ip=&i;
lp=&l;
dp=&d;
pcp=&cp;
pfp=&fp;
pip=&ip;
plp=&lp;
pdp=&dp;
printf("Data type Address of pointer Address of value Value\n");
printf(" **p \t *p \t p\n");
printf("Character %8x %10x %c\n", pcp, *pcp, **pcp);
printf("Float %8x %10x %f\n", pfp, *pfp, **pfp);
printf("Integer %8x %10x %d\n", pip, *pip, **pip);
printf("Long %8x %10x %ld\n", plp, *plp, **plp);
printf("Double %8x %10x %lf\n", pdp, *pdp, **pdp);
printf("After increment\nData type Address of pointer Address of garbage Garbage value\n");
printf(" **p \t *p \t p\n");
cp++;
fp++;
ip++;
lp++;
dp++;
printf("Character %8x %10x %c\n", pcp, *pcp, **pcp);
printf("Float %8x %10x %f\n", pfp, *pfp, **pfp);
printf("Integer %8x %10x %d\n", pip, *pip, **pip);
printf("Long %8x %10x %ld\n", plp, *plp, **plp);
printf("Double %8x %10x %lf\n", pdp, *pdp,- **pdp);
}
OUTPUT:
Data type Address of pointer Address of value Value
**p *p p
Character 61ff04 61ff08 S
Float 61fefc 61ff00 12.500000
Integer 61fef4 61fef8 486
Long 61feec 61fef0 517
Double 61fedc 61fee0 10.990000
After increment
Data type Address of pointer Address of garbage Garbage value
**p *p p
Character 61ff04 61ff0c ▄
Float 61fefc 61ff04 0.000000
Integer 61fef4 61fefc 6422276
Long 61feec 61fef4 6422268
Double 61fedc 61fee8 -0.000000
Calculate perimeter to rectangle.
#include <stdio.h>
void main()
{
int *len, *br, *perim;
len=(int*)malloc(sizeof(int));
br=(int*)malloc(sizeof(int));
perim=(int*)malloc(sizeof(int));
printf("Enter length\n");
scanf("%d", len);
printf("Enter breadth\n");
scanf("%d", br);
*perim=2*(*len+*br);
printf("Length = %d\n", *len);
printf("Breadth = %d\n", *br);
printf("Perimeter of rectangle= %d", *perim);
}
Output:
Enter length
4
Enter breadth
4
Length = 4
Breadth = 4
Perimeter of rectangle= 16
Solve following expression: (a2 + b2 )2
#include <stdio.h>
void main()
{
int *a, *b, *s;
a=(int*)malloc(sizeof(int));
b=(int*)malloc(sizeof(int));
s=(int*)malloc(sizeof(int));
printf("Enter value of a\n");
scanf("%d", a);
printf("Enter value of b\n");
scanf("%d", b);
*s=(*a * *a)+(*b * *b);
printf("a = %d\n", *a);
printf("b = %d\n", *b);
printf("Answer = %d", *s);
}
Output:
Enter value of a
5
Enter value of b
6
a = 5
b = 6
Answer = 61
Find area and circumference of circle.
#include <stdio.h>
void main()
{
int *rad;
const float pi=3.1414;
float *ar, *cf;
rad=(int*)malloc(sizeof(int));
ar=(int*)malloc(sizeof(int));
cf=(int*)malloc(sizeof(int));
printf("Enter radious\n");
scanf("%d", rad);
*ar=pi * *rad * *rad;
*cf=2 * pi * *rad;
printf("Area = %f\n", *ar);
printf("Circumference = %f", *cf);
}
Output:
Enter radious
4
Area = 50.262402
Circumference = 25.131201
C Program to show array using pointer.
#include <stdio.h>
void main()
{
int p, n, *pp;
printf("Enter number of elements: \n");
scanf("%d", &n);
pp=(int*)malloc(sizeof(int)*n);
for(p=0;p<n;p++)
{
printf("%dth element: \n", p+1);
scanf("%d", &pp[p]);
}
printf("\n");
for(p=0;p<n;p++)
printf("Element%d: %d", p+1, pp[p]);
}
OUTPUT:
Enter number of elements:
4
1th element:
2
2th element:
5
3th element:
4
4th element:
2
Element1: 2
Element2: 5
Element3: 4
Element4: 2
C Program to show reverse array using pointer.
#include <stdio.h>
void main()
{
int p, n, *pp;
printf("Enter number of elements: \n");
scanf("%d", &n);
pp=(int*)malloc(sizeof(int)*n);
for(p=0;p<n;p++)
{
printf("%dth element: \n", p+1);
scanf("%d", pp);
pp++;
}
printf("\n");
pp--;
for(p=n-1;p>=0;p--)
{
printf("Element%d: %d\n", p+1, *pp);
pp--;
}
}
OUTPUT:
Enter number of elements:
5
1th element:
1
2th element:
2
3th element:
3
4th element:
4
5th element:
5
Element5: 5
Element4: 4
Element3: 3
Element2: 2
Element1: 1
Write a C Program to add n numbers.
#include <stdio.h>
void main()
{
int p, n, *pp, add=0;
printf("Enter number of elements: \n");
scanf("%d", &n);
pp=(int*)malloc(sizeof(int)*n);
for(p=0;p<n;p++)
{
printf("%dth element: \n", p+1);
scanf("%d",&pp+p);
add+=(*(pp+p));
}
printf("\n");
for(p=n-1;p>=0;p--)
{
printf("Element%d: %d\n", p+1, *(pp+p));
}
printf("Addition = %d", add);
}
OUTPUT:
Enter number of elements:
5
1th element:
5
2th element:
4
3th element:
6
4th element:
5
5th element:
4
Element5: 4
Element4: 5
Element3: 6
Element2: 4
Element1: 5
Addition = 24
Program to demonstrate 2D array using pointer.
#include <stdio.h>
void main()
{
int p, q, c, r, **pp, add;
float avg;
printf("Enter number of columns and rows: \n");
scanf("%d%d", &c, &r);
pp=(int**)malloc(sizeof(int)*c);
for(p=0;p<c;p++)pp[p]=(int*)malloc(sizeof(int)*r);
printf("\n");
for(p=0;p<c;p++)
for(q=0;q<r;q++)
{
printf("Element[%d][%d]\n", p+1, q+1);
scanf("%d",&pp[p][q]);
add+=pp[p][q];
}
for(p=0;p<c;p++)
{
for(q=0;q<r;q++)
printf("\t%d", pp[p][q]);
printf("\n");
}
avg=(float)add/(c*r);
printf("Average = %f", avg);
}
Output:
Enter number of columns and rows:
3 3
Element[1][1]
11
Element[1][2]
22
Element[1][3]
33
Element[2][1]
44
Element[2][2]
55
Element[2][3]
66
Element[3][1]
77
Element[3][2]
88
Element[3][3]
99
11 22 33
44 55 66
77 88 99
Average = 55.000000
Program to demonstrate pointer to constant declaration.
#include <stdio.h>
void main()
{
int p= 49;
const int *ptr;
ptr=&p;
printf("Address of p = %u\n", ptr);
printf("Value of p = %d", p);
getch();
}
OUTPUT:
Address of p = 6422296
Value of p = 49