Character input and output functions in C
This stdio.h header file provides built-in functions for reading and writing characters from inputs and output devices. Thus, they are getchar() and putchar() functions. They at a time read or print one character.
getchar() function: This function is use to accept one character from the keyboard, and this may be any character from C character set(i.e it should be a printable or non-printable characters).
putchar() function :
This function is use to print one character on the screen, and this may be any character from C character set (i.e. it may be printable or non printable characters).
getch() function: The getch() function is use to get characters from the console that is from the keyboard without echo to the screen.
What are string input and output functions in C?
The stdio.h header file provides built-in functions for reading and writing strings from input-output devices. They are gets() and puts() functions.
gets() function: This function is used to accept a string from the keyboard, it continues reading character by character till “Enter key is pressed” and newline character ‘\n’ is replaced with the NULL character ‘\0’.
puts() function: This function is used to print a string on the screen, It also appends newline (i.e. ‘\n’) character at the end of the string.
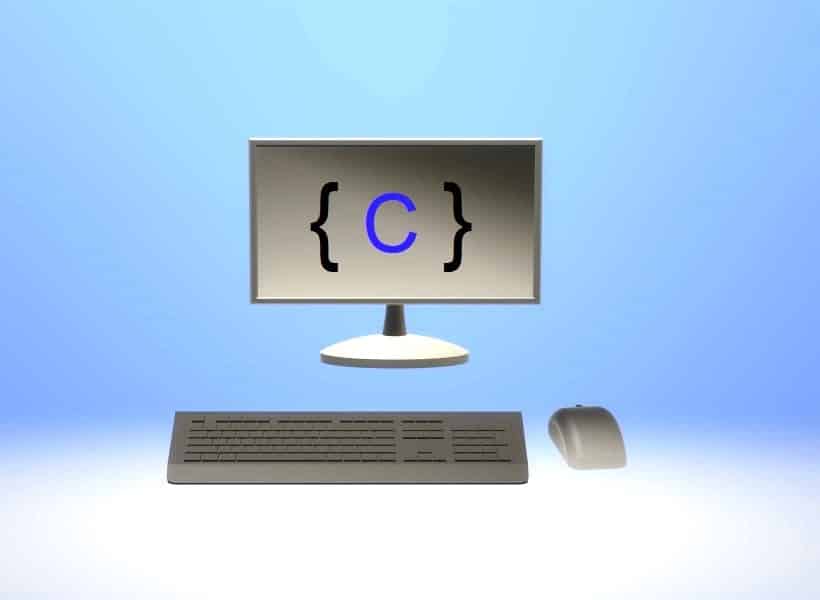
Program to convert hexadecimal number to its octal equivalent.
#include <stdio.h>
int main()
{
int h, o;
printf("Enter hexadecimal number:\n");
scanf("%x",&h);
printf("Hexadecimal number: %x\n", h);
printf("Octal equivalent: %o\n", h);
return 0;
}
Output:
Enter hexadecimal number: B5
Hexadecimal number: b5
Octal equivalent: 265
Program to convert distance in centimeter to feet and inches.
#include <stdio.h>
void main()
{
float c, f, i;
printf("Enter distance in centimeter:\n");
scanf("%f",&c);
f = c/30;
i = c/1.25;
printf("Distance in centimeter: %f\n", c);
printf("Distance in feet: %f\n", f);
printf("Distance in inches: %f", i);
}
Output:
Enter distance in centimeter:
Distance in centimeter: 146.000000
Distance in feet: 4.866667
Distance in inches: 116.800003
C Program to calculate the area of a triangle.
#include<stdio.h>
#include<math.h>
void main()
{
int a, b, c, perim;
double area;
printf("Enter the three sides of the triangle: \n");
scanf("%d %d %d", &a, &b, &c);
areaTri(a, b, c, &perim, &area);
printf("Sides of triangle = %d %d %d\n", a, b, c);
printf("Perimeter of triangle = %d\n", perim);
printf("Area of triangle = %lf", area);
}
void areaTri(int a, int b, int c, int *p, double *ar)
{
double s;
*p=a+b+c;
s=*p/2;
*ar=pow((s*(s-a)*(s-b)*(s-c)),(0.5));
}
OUTPUT:
Enter the three sides of the triangle:
10 20 15
Sides of triangle = 10 20 15
Perimeter of triangle = 45
Area of triangle = 60.794737