A string is an array of characters stored in consecutive memory locations. Therefore, In strings, the ending character is always the null character ‘\0’. Hence, The null character acts as a string terminator. However, you get various types of string-based programs. Therefore, You can learn more Strings C program.
Standard Library for String Functions
#include<string.h>: Although, This header file contains many functions among them some are listed below:
- strlen(s1): Returns length of string s1.It excludes a null character that is ‘\0’.
- strlwr(s1): It converts the string s1 to lowercase.
- strcat(s1,s2): Inserts a copy of string s2 to the end of s1 and dump s1 with a null and returns s1.
- strcmp(s1,s2): This function compares s1 and s2 and returns negative if s1<s2, positive if s1>s2, 0 if s1=s2.
- strcpy(s1,s2): It copies the s2 string into s1 string, and modify the s1 string.
- strupr(s1): It converts the string s1 into uppercase.
- strstr(s1,s2): This function returns a pointer to the first occurrence of the s2 string in the s1 string.
- strrev(s1): It returns the reverse string of the s1 string.
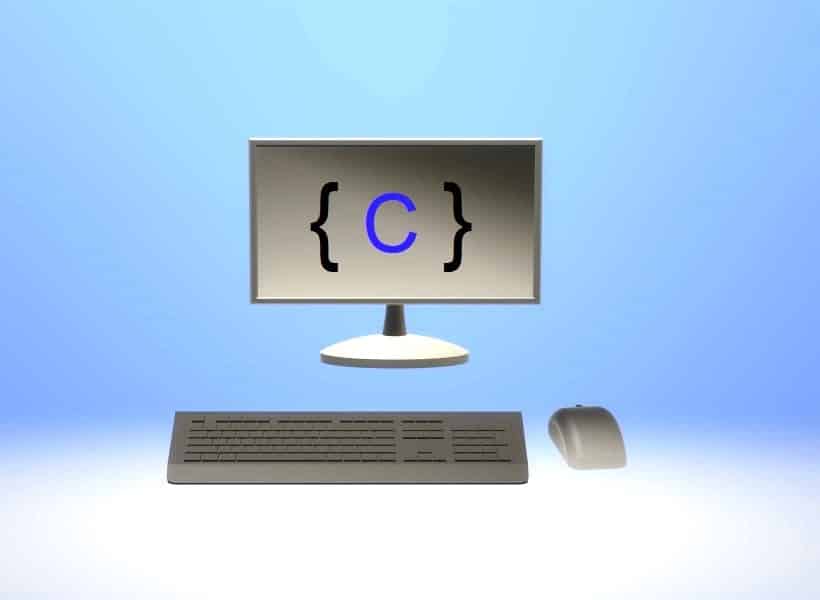
Accept a C Program for concatenating the strings.
#include <stdio.h>
#include <string.h>
void main()
{
char str1[50], str2[50];
puts("Enter the string 1: \n");
gets(str1);
puts("Enter the string 2: \n");
gets(str2);
printf("The string 1 is: %s\n", str1);
printf("The string 2 is: %s\n", str2);
strcat(str1, str2);
printf("The string 3 is: %s", str1);
}
OUTPUT:
Enter the string 1:
code
Enter the string 2:
forever
The string 1 is: code
The string 2 is: forever
The string 3 is: codeforever
C Program to copy the strings
#include <stdio.h>
#include <string.h>
void main()
{
char str1[50], str2[50];
puts("Enter the string 1: ");
gets(str1);
puts("Enter the string 2: ");
gets(str2);
printf("The string 1 is: %s\n", str1);
printf("The string 2 is: %s\n", str2);
strcpy(str2, str1);
printf("Copied strings are: \n");
printf("The string 1 is: %s\n", str1);
printf("The string 2 is: %s", str2);
}
OUTPUT:
Enter the string 1:
Code
Enter the string 2:
Forever
The string 1 is: Code
The string 2 is: Forever
Copied strings are:
The string 1 is: Code
The string 2 is: Code
Write C Program to separate words from a string(using sscanf()).
#include <stdio.h>
void main()
{
char str[50], tok[20][40];
int p, tkn;
puts("Enter the string: \n");
gets(str);
tkn=sscanf(str, "%s%s%s%s%s%s%s%s", tok[0], tok[1], tok[2], tok[3], tok[4], tok[5], tok[6], tok[7]);
printf("\nLine has %d words\n", tkn);
for(p=0;p<tkn;p++)
printf("%s \n", tok[p]);
}
OUTPUT:
Enter the string:
Code Forever is the best.
Line has 5 words
Code
Forever
is
the
best.
C Program to separate words from a string (use user-defined function).
#include <stdio.h>
void main()
{
char str[50], tok[20][50];
int p, tkn;
puts("Enter the string: \n");
gets(str);
tkn=words(str, tok, 20, 50);
printf("\nLine has %d words\n", tkn);
for(p=0;p<tkn;p++)
printf("%s", tok[p]);
}
int words(char *str, char w[][50], int x, int y)
{
int p=0, q, r=0;
do
{
q=0;
do
{
w[p][q]=str[r];
if(str[r]=='\0')
break;
r++;
q++;
}
while(str[r]!=' ');
while(str[r]==' ')
r++;
w[p][q]='\0';
p++;
}
while(str[r]!='\0');
return(p);
}
Output:
Enter the string:
Code Forever is the best
Line has 5 words
CodeForeveristhebest
Accept a string and find out the occurrences of each vowel in it.
#include<stdio.h>
void count(char *d)
{
int a=0,e=0,i=0,o=0,u=0,k=0;
do
{
switch(d[k])
{
case 'a':
case 'A': a++;
break;
case 'e':
case 'E': e++;
break;
case 'i':
case 'I': i++;
break;
case 'o':
case 'O': o++;
break;
case 'u':
case 'U': u++;
break;
}
k++;
}while(d[k]!='\0');
printf("\nTotal number of a or A found: %d",a);
printf("\nTotal number of e or E found: %d",e);
printf("\nTotal number of i or I found: %d",i);
printf("\nTotal number of o or O found: %d",o);
printf("\nTotal number of u or U found: %d",u);
}
void main()
{
char str[50];
puts("Enter the string 1: ");
gets(str);
printf("\n The string is %s", str);
count(str);
}
Output:
Enter the string 1:
Code Forever
The string is Code Forever
Total number of a or A found: 0
Total number of e or E found: 3
Total number of i or I found: 0
Total number of o or O found: 2
Total number of u or U found: 0
Write a C program to display the occurrence of a substring in the string.
#include<stdio.h>
int count(char *d,char *s,int *p)
{
int cnt,i=0,j=0,temp;
*p=-1;
do
{
if(d[i]==s[j])
{
temp=i+1;
do
{
if(d[i]!=s[j])
break;
i++;
j++;
}while(s[j]!='\0');
if(s[j]=='\0')
{
if(*p==-1)*p=temp;
cnt++;
j=0;
}
i--;
}
i++;
}while(d[i]!='\0');
return(cnt);
}
void main()
{
char str[50],temp[50];
int pos, s;
puts("Enter the First string ");
gets(str);
puts("\nEnter the Second string ");
gets(temp);
s=count(str,temp,&pos);
printf("\nString 1: %s",str);
printf("\nString 2: %s",temp);
printf("\nOccurrence of String 2 in String 1: %d",s);
printf("\nFirst occurrence found at position: %d",pos);
}
Output:
Enter the First string
Code Forever provides various tutorial for programming languages
Enter the Second string
for
String 1: Code Forever provides various tutorial for program
String 2: for
Occurrence of String 2 in String 1: 2
First occurrence found at position: 40
Write a program to insert a number of characters of substring at a given position in the string.
#include<stdio.h>
char * insert_str(char *d,char *s,int p,int l)
{
int i=0,j=0,k=0;
char *c;
c=(char*)malloc(sizeof(char)*80);
do
{
c[k]=d[i];
k++;i++;
if(i>=p-1)
break;
}while(d[i]!='\0');
do
{
c[k]=s[j];
k++;j++;
if(j>=l)
break;
}while(s[j]!='\0');
if(p!=i)
{
do
{
c[k]=d[i];
k++; i++;
}while(d[i]!='\0');
c[k]='\0';
}
return(c);
}
void main()
{
char str[50],s2[50],*s3;
int pos,l;
puts("Enter the First string");
gets(str);
puts("\nEnter the second string");
gets(s2);
printf("\nEnter the position: ");
scanf("%d",&pos);
printf("\nEnter the length: ");
scanf("%d",&l);
s3=insert_str(str,s2,pos,l);
printf("\nString 1: %s",str);
printf("\nString 2: %s",s2);
printf("\nString 3: %s",s3);
}
Output:
Enter the First string
CodeForever
Enter the second string
best
Enter the position: 4
Enter the length: 3
String 1: CodeForever
String 2: best
String 3: CodbeseForever
Program to display surname with initials
#include<stdio.h>
void main()
{
char str[50], tok[20][50];
int p, tkn;
puts("Enter the string: \n");
gets(str);
tkn=words(str, tok);
printf("Name\n %s", str);
printf("Name with initials: %s %c. %c", tok[2], tok[0][0], tok[1][0]);
}
int words(char *str, char w[][50])
{
int p=0, q, r=0;
do
{
q=0;
do
{
w[p][q]=str[r];
if(str[r]=='\0')
break;
r++;
q++;
}
while(str[r]!=' ');
while(str[r]==' ')
r++;
w[p][q]='\0';
p++;
}
while(str[r]!='\0');
return(p);
}
Output:
Enter the string:
Code Forever
Name
Code ForeverName with initials: │ C. F
C program to convert String into the case
#include<stdio.h>
void change_case(char *des)
{
int l=0,a=0,e=0,i=0,o=0,u=0;
do
{
if((des[l]>=65)&&(des[l]<=90))
des[l]+=32;
else if((des[l]>=97) && (des[l]<=122))
des[l]-=32;
l++;
}while(des[l]!='\0');
}
void main()
{
char s[50];
puts("Enter the string 1\n");
gets(s);
printf("The given string: %s",s);
change_case(s);
printf("\n The converted string: %s",s);
}
Output:
Enter the string 1
Code fOrever
The given string: Code fOrever
The converted string: cODE FoREVER
Accept a String convert into Upper and lower case.
#include<stdio.h>
void caseCapi(char *dest)
{
int j=0,a=0,e=0,i=0,o=0,u=0;
do{
if((dest[j]>=97)&&(dest[j]<=122))
{
dest[j]-=32;
}
j++;
}while(dest[j]!='\0');
}
void caseSmall(char *dest)
{
int j=0,a=0,e=0,i=0,o=0,u=0;
do{
if((dest[j]>=65)&&(dest[j]<=90))
{
dest[j]+=32;
}
j++;
}while(dest[j]!='\0');
}
void main()
{
char str[50];
puts("\nEnter the string 1: ");
gets(str);
printf("\nThe Given String: %s",str);
caseCapi(str);
printf("\nThe Uppercase String: %s",str);
caseSmall(str);
printf("\nThe lowercase String: %s",str);
}
Output:
Enter the string 1:
Code ForEvEr
The Given String: Code ForEvEr
The Uppercase String: CODE FOREVER
The lowercase String: code forever
Write C program to check palindrome
#include<stdio.h>
int palindrome(char *dest)
{
int j=0,k,i,flag=1;
do
{
j++;
}while(dest[j]!='\0');
k=j-1;i=0;
do
{
if(dest[i]!=dest[k]){flag=0;break;}
i++;k--;
}while(i<=k);
return(flag);
}
void main()
{
char str[50];
puts("Enter the String");
gets(str);
printf("\nGiven Sting : %s",str);
if(palindrome(str))
printf("\nIt is Palindrome");
else
printf("\nIt is not Palindrome");
}
Output:
Enter the String
Code FOrever
Given Sting : Code FOrever
It is not Palindrome
Write C Program to encrypt and decrypt the string
#include<stdio.h>
void encrypt(char *dest)
{
int j=0;
do{
dest[j]-=20;
j++;
}while(dest[j]!='\0');
}
void decrypt(char *dest)
{
int j=0;
do
{
dest[j]+=20;
j++;
}while(dest[j]!='\0');
}
void main()
{
char str[80];
puts("Enter the String: ");
gets(str);
printf("\nThe Given String: %s",str);
encrypt(str);
printf("\nEncrypted String: %s",str);
decrypt(str);
printf("\nDecrypted String: %s",str);
}
Output:
Enter the String:
Code Forever
The Given String: Code Forever
Encrypted String: /[PQ2[^QbQ^
Decrypted String: Code Forever